How To Master Advanced JSON Querying in SQLite
TLDR; This comprehensive guide explores the power and capabilities of JSON in SQLite, shedding light on SQLite's JSON functions and how they can be used to work with JSON data in SQLite, through step-by-step SQL query examples. We've covered advanced querying techniques for hierarchical JSON data, schema validation, and leveraging indexing for query optimization. Finally, we explained some common pitfalls to avoid when querying JSON data in SQLite, along with troubleshooting tips. Overall, it's a deep dive into SQLite's JSON capabilities, offering valuable insights and practical approaches for programmers dealing with JSON data in SQLite.
In the prior article, Learning the Basics: How to Use JSON in SQLite, we dived into SQLite's essential JSON functions and their capabilities. We explored the use of JSON as unstructured data within an SQLite database. Crucially, we detailed some of the necessary SQLite JSON functions, discussing their role in data storage and retrieval, followed by practical SQL query examples. This foundational understanding of how to work with JSON data in SQLite sets the stage for your advanced exploration of the topic.
Let's get started!
Integrating SQL and NoSQL Capabilities Through Fully Grasping JSON Handling in SQLite
Advancing your knowledge about SQLite's JSON handling capabilities combines the best of SQL and NoSQL, providing an efficient, all-in-one solution for managing mixed data formats. JSON data support in SQLite turns SQLite into a powerhouse for unstructured data, similar to databases like MongoDB.
SQLite's advanced JSON integration brings together JSON's flexibility and SQLite's robustness, ideal for today's data-heavy applications. SQLite's JSON capabilities do more than just store and retrieve data. They allow for SQL-like operations on JSON data, creating a bridge between structured and unstructured data management.
This guide focuses on filing your practical skill sets with SQLite's JSON functions through hands-on SQL query examples. Each section is intended to boost your understanding and give you a head start over real-world JSON data manipulation in SQLite.
By the end, you'll be well-equipped with the available toolset of JSON data handling in SQLite to tackle any JSON data structures. You’ll be learning about how to apply indexes, query with path expressions, filter, and even validate data—essential tasks for handling dynamic data in structured environments using JSON functions in SQLite.
Table of Contents
- Section 1: How To Integrate JSON Within SQLite?
- Section 2: Leveraging SQLite JSON Functions for Advanced JSON Decoding and SQL Querying
- Section 3: Practical Approaches To Query Any Complex JSON Data in SQLite
- Section 4: How To Check the Schema of Your JSON Data in SQLite?
- Section 5: How To Manage Nested JSON Data in SQLite
- Section 6: How To Use Indexing for Query Optimization Over JSON Data in SQLite?
- Section 7: Json5 Support in SQLite
- Section 8: Common Mistakes and Troubleshooting When Working With JSON in SQLite
Section 1: How To Integrate JSON Within SQLite?
SQLite's built-in JSON functions play a pivotal role in integrating JSON and SQLite. As of SQLite version 3.38.0, released in 2022-02-22 onwards, JSON functions are included by default, whereas before they were an extension. This means that before this version these JSON functions in SQLite were opt-in, whereas now they are available by default and can be opt-out by setting a compile-time option, in case you need to disable them.
You can import JSON data into SQLite using simple insert SQL queries. Alternatively, you can also utilize third-party tools or scripting techniques to bulk import extensive JSON datasets as well. To extract JSON data, you can leverage the json_extract() function that fetches values linked to a specific key from a JSON data column.
Section 2: Leveraging SQLite JSON Functions for Advanced JSON Decoding and SQL Querying
In this section we are going to explore advanced JSON functions and their capabilities in SQLite, using SQL query examples for each one. Throughout this blog post, we are going to use sample generated JSON data, named movie as a reference to be used as examined data:
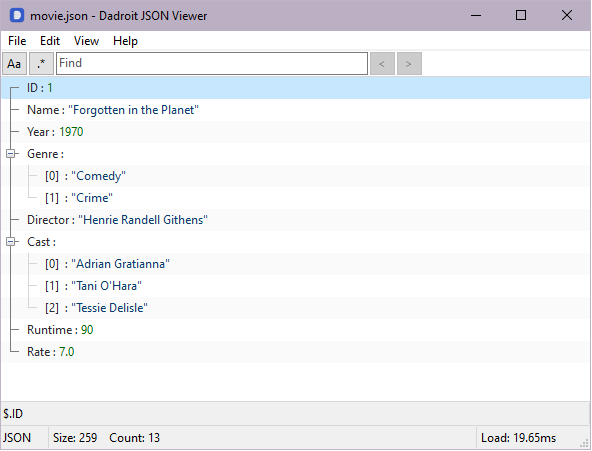
You can insert the data into a table named movie with one field named data, and start running these sample queries from now on against it. In the following queries, we are going to use the input texts of JSON functions, to be straightforward about the explanation of the functions, and then we’ll get back to the data inserted in the database starting from section 3.
For the sake of simplicity in this example, we are going to use a simpler version of the first JSON data:
{
"Name": "Naked of Truth",
"Year": 1979,
"Director": "Ellynn O'Brien",
"Producer": "Kayley Byron Tutt",
"Runtime": 183,
"Rate": 8.0,
"Description": "Donec pretium nec dolor in auctor."
}
Error Detection With json_error_position()
Function in SQLite
The json_error_position() function can be used to detect any error in the syntax of your JSON data. If the input string is a valid JSON it will return 0, otherwise, it will return the character position of the first error.
For example, if you have a broken JSON string as the input of this function like this:
SELECT
json_error_position ( '{"Name":"Naked of Truth","Year":1979,' ) AS err_position
The result of running this query would be the position of error syntax that occurred, which in this case is the position of missing “}” at the end:
error_position |
---|
38 |
Merge JSON Objects With json_patch()
Function in SQLite
The json_patch() function merges 2 JSON objects, allowing to add, modify, and delete JSON objects.
For example, this query would combine the 2 JSON inputs into 1 JSON:
SELECT
json_patch ( '{"Name":"Naked of Truth"}', '{"Year": 2011}' ) AS patched_json;
The result would be something like this, a JSON object constructed of both of the fields:
patched_json |
---|
{"Name":"Naked of Truth","Year":2011} |
Manipulate JSON Fields by Using the json_set()
Function in SQLite
The json_set() function is used to add or replace JSON properties. json_set()
takes a JSON string as its first argument followed by zero or more pairs of path/value arguments. The result would be a JSON string created from adding or replacing values based on the provided path and value pairs.
For example, building on the previous query’s JSON data, if you want to append a Director
field to the JSON data, you can write a query like this:
SELECT
json_set ( '{"Name":"Naked of Truth","Year":2011}', '$.Director', 'Ellynn OBrien' ) AS json_data;
And the result would be something like this:
json_data |
---|
{"Name":"Naked of Truth","Year":2011,"Director":"Ellynn OBrien"} |
The json_quote()
Function in SQLite
The json_quote() function is a simple one, it just wraps the input value with double quotes to make it a valid JSON string. Here is a simple query example of it:
SELECT
json_quote ( 'Naked Of Truth' ) AS valid_json_string;
And the result would be something like this:
valid_json_string |
---|
"Naked of Truth" |
How To Use json_group_object()
and json_group_array()
JSON Functions in SQLite for Aggregation
For this set of JSON functions in SQLite, we need to expand the sample JSON data in comparison with the previous examples, to demonstrate the use case of each function in an understandable way. Suppose this is your movie
table in the database with one field named data
, as mentioned at the start of this section:
data |
---|
{"ID": 1, "Name": "Forgotten in the Planet", "Year": 1970, "Genre": ["Comedy", "Crime"], "Director": "Henrie Randell Githens", "Cast": ["Adrian Gratianna", "Tani O'Hara", "Tessie Delisle"], "Runtime": 90, "Rate": 7.0} |
{"ID": 2, "Name": "The Obsessed's Fairy", "Year": 1972, "Genre": ["Adventure"], "Director": "Susanne Uriel Lorimer", "Cast": ["Dacy Dex Elsa", "Matilde Kenton Collins"], "Runtime": 98, "Rate": 9.5} |
{"ID": 3, "Name": "Last in the Kiss", "Year": 1965, "Genre": ["History", "Animation"], "Director": "Simone Mikey Bryn", "Cast": ["Margery Maximilianus Shirk","Harri Garwood Michelle"], "Runtime": 106, "Rate": 4.1} |
The json_group_array()
Aggregate Function With SQL Query Example
The json_group_array() function similar to any other aggregate function in SQLite, groups multiple rows of data into a single JSON array.
For example, this query would return a JSON array with all the names of the movies with a Rate bigger than 6:
SELECT
json_group_array ( json_extract ( data, '$.Name' ) ) AS movie_names
FROM
movie
WHERE
json_extract ( data, '$.Rate' ) > 6
And the result would be something like this:
movie_names |
---|
["Forgotten in the Planet", "The Obsessed's Fairy"] |
The json_group_object()
JSON Function With SQL Query Example
The json_group_object() function creates a JSON object by grouping two columns of a query, where the first column is used as the key, and the second as the value. The first will be used as the key name of the JSON fields, and the second as their values.
For example, this query will return a JSON object where each field’s name is a movie's ID and the field’s value is the corresponding Name if the movie
has a Rate bigger than 6, which would exclude the last movie:
SELECT
json_group_object ( json_extract ( Data, '$.ID' ), json_extract ( Data, '$.Name' ) ) AS movie_rates
FROM
movie
WHERE
json_extract ( Data, '$.Rate' ) > 5
The result would be something like this, a JSON object consisting of the ID and Name of the first and second movies because they have a Rate
greater than 5:
movie_rates |
---|
{"1": "Forgotten in the Planet","2":"The Obsessed's Fairy"} |
Parse JSON Data With json_each()
and json_tree()
Table-Valued Functions in SQLite
SQLite offers two powerful table-valued functions to work with your JSON data, json_each()
and json_tree()
. They have variations with and without the path parameter, allowing you to interact with your JSON at different depths.
Suppose this is your only JSON value inserted in the data field of the movie table in the SQLite database, let’s start explaining the aggregate functions upon it:
data |
---|
{ "ID": 1, "Name": "Forgotten in the Planet", "Year": 1970, "Genre": ["Comedy", "Crime"], "Director": "Henrie Randell Githens", "Cast": ["Adrian Gratianna", "Tani O'Hara", "Tessie Delisle"], "Runtime": 90, "Rate": 7.0 } |
The json_each()
Function in SQLite With SQL Query Example
The json_each() function breaks a JSON object into rows, with each row representing a field in the JSON object, going only through level 1 of nested JSON fields.
For example, this query would return 8 rows for each field in the JSON data:
SELECT
key,
value,
type
FROM
movie,
json_each ( data )
The result would be something like this, listing the key and values of each field in the JSON as a row, As you see, the array field Genre
and Cast
are listed as they are, and the function did not go into them to list the second level items:
key | Value | Type |
---|---|---|
ID | 1 | integer |
Name | Forgotten in the Planet | text |
Year | 1970 | integer |
Genre | ["Comedy","Crime"] | array |
Director | Henrie Randell Githens | text |
Cast | ["Adrian Gratianna","Tani O'Hara","Tessie Delisle"] | array |
Runtime | 90 | integer |
Rate | 7.0 | real |
The json_tree()
Function in SQLite With SQL Query Example
The json_tree() function is used to traverse and parse JSON data completely, meaning it would go into each field through all the nested levels. The json_tree()
function goes through the JSON, examining every part of it, and then gives you a table that details every element it found.
The json_tree()
displays the results as a set of rows, providing a clear view of even the most complex nested JSON data. This table tells you the name of each element, what type of data it is, its value, and where it is located within the JSON structure.
So This query would return several rows, describing the structure of the JSON object, including the nested Cast field:
SELECT
key,
value,
type
FROM
movie,
json_tree ( data )
The result of the above query would be something like this:
key | Value | Type |
---|---|---|
{"ID":1,"Name":"Forgotten in the Planet","Year":1970,"Genre":["Comedy","Crime"],"Director":"Henrie Randell Githens","Cast":["Adrian Gratianna","Tani O'Hara","Tessie Delisle"],"Runtime":90,"Rate":7.0} | object | |
ID | 1 | integer |
Name | Forgotten in the Planet | text |
Year | 1970 | integer |
Genre | ["Comedy","Crime"] | array |
0 | Comedy | text |
1 | Crime | text |
Director | Henrie Randell Githens | text |
Cast | ["Adrian Gratianna","Tani O'Hara","Tessie Delisle"] | array |
0 | Adrian Gratianna | text |
1 | Tani O'Hara | text |
2 | Tessie Delisle | text |
Runtime | 90 | integer |
Rate | 7 | real |
With the path parameter, json_tree()
can focus on a specific part of the JSON. If you give json_tree()
a specific path in the JSON as the second argument, it will start its exploration from there.
For example, this query ignores everything outside the Cast field, offering a focused view of this nested JSON array:
SELECT
key,
value,
type
FROM
movie,
json_tree ( data, '$.Cast' )
The result of the above query would be something like this:
key | Value | Type |
---|---|---|
0 | Adrian Gratianna | text |
1 | Tani O'Hara | text |
2 | Tessie Delisle | text |
Fun fact: Have you ever noticed the '1' in the URL of the official documentation of JSON in SQLite and wondered if it has a story? When JSON support was first released in SQLite back in 2015, the creator expected that 'JSON1' would be just the beginning of a series of versions—JSON2, JSON3, and so on. But here's the fun part: 'JSON1' was so effective and efficient that they never had to create a 'JSON2' or 'JSON3'. So, the '1' in 'JSON1' isn't merely a version indicator—it's a mark of success!
Section 3: Practical Approaches To Query Any Complex JSON Data in SQLite
Using SQLite's JSON functions in collaboration with SQLite’s built-in functions allows you to perform more complex data querying. Here you can see some of these examples including aggregation, filtering, and path expressions.
As mentioned at the beginning of the post, the JSON data in the movie
table in the examples for all of the remained sections would be like this:
data |
---|
{"ID": 1, "Name": "Forgotten in the Planet", "Year": 1970, "Genre": ["Comedy", "Crime"], "Director": "Henrie Randell Githens", "Cast": ["Adrian Gratianna", "Tani O'Hara", "Tessie Delisle"], "Runtime": 90, "Rate": 7.0} |
{"ID": 2, "Name": "The Obsessed's Fairy", "Year": 1972, "Genre": ["Comedy", "Adventure"], "Director": "Susanne Uriel Lorimer", "Cast": ["Dacy Dex Elsa", "Matilde Kenton Collins"], "Runtime": 98, "Rate": 9.5} |
{"ID": 3, "Name": "Last in the Kiss", "Year": 1965, "Genre": ["History", "Animation"], "Director": "Simone Mikey Bryn", "Cast": ["Margery Maximilianus Shirk","Harri Garwood Michelle"], "Runtime": 106, "Rate": 4.1} |
Crafting Aggregate SQL Queries With JSON Functions in SQLite
This approach involves using JSON functions along with SQLite's built-in aggregate functions to perform calculations on JSON data. For example, you can calculate the average Runtime of the movie categorized as a Comedy by using the following query:
SELECT
AVG( json_extract ( data, '$.Runtime' ) ) AS average_runtime
FROM
movie AS M,
json_each ( json_extract ( M.data, '$.Genre' ) ) AS T
WHERE
T.value = 'Comedy';
The result of the above query would be something like this since there are 2 movies in the database with the Comedy genre, and their Runtime is 90 and 98, so the average of them would be like this:
average_runtime |
---|
94 |
JSON Decoding and Filtering Data With Multiple Conditions
You can utilize the json_extract()
function in SQLite for in-depth filtering by using it in the WHERE
clause of an SQL query. For example, you can filter movies based on specific conditions, such as the movies that have two Cast members or more, and a Rate
higher than a certain value.
SELECT
json_extract ( data, '$.Name' ) AS movie_name,
json_extract ( data, '$.Rate' ) AS movie_rate,
json_array_length ( json_extract ( data, '$.Cast' ) ) AS cast_size
FROM
movie
WHERE
json_array_length ( json_extract ( data, '$.Cast' ) ) >= 2
AND json_extract ( data, '$.Rate' ) > 8;
The result of the above query would be something like this:
movie_name | movie_rate | cast_size |
---|---|---|
The Obsessed's Fairy | 9.5 | 2 |
Using Path Expressions To Extract Specific Values From JSON Data in SQLite
Path expressions can be used to access nested JSON data at that specific address. This example returns a list of all movie
directors
who directed a movie in a certain Genre, like History.
SELECT DISTINCT
json_extract ( data, '$.Director' ) AS movie_director
FROM
movie,
json_each ( json_extract ( data, '$.Genre' ) )
WHERE
value = 'History';
The result of the above query would be something like this:
movie_director |
---|
Simone Mikey Bryn |
Section 4: How To Check the Schema of Your JSON Data in SQLite?
Schema checking of JSON data in SQLite is a way to ensure the structure and consistency of your data, improve future error handling, and simplify complex data manipulation. Although SQLite lacks built-in functions for schema validation, you can use its JSON and the CHECK function for this purpose.
Checking JSON Structure With json_type()
and check()
SQLite Functions
The json_type()
function can be used to check the type of a field in the JSON data. For example, building on the previous creation of the movie table, suppose when creating the table to store a movie’s JSON data, you want to ensure that every entry has the Name and Year fields, with Year being an integer. For that, you can use a CHECK() constraint with the json_type()
function in the table creation:
CREATE TABLE movie ( data TEXT CHECK ( json_type ( data, '$.Name' ) IS NOT NULL AND json_type ( data, '$.Year' ) = 'integer' ) );
Here json_type()
checks the type of the specified fields in your JSON data, the Name, and the Year. If a new insertion or update operation tries to add data where Name does not exist or Year is not an integer, the CHECK() constraint will fail and the operation will be rejected. This helps maintain the data integrity of your JSON data in the movie table.
Validating JSON Data Using the json_valid()
Function in SQLite
The json_valid()
function verifies the validity of the JSON data from the JSON standard format perspective, offering a degree of schema validation. For example, to ensure the integrity of the JSON data before insertion, you can apply validation checks like this:
INSERT INTO movie ( data ) SELECT
'{"Name":"Naked of Truth","Year":1979}' AS movie_input
WHERE
json_valid ( movie_input );
In this statement, json_valid()
checks whether the provided JSON string is valid. If it is, the data is inserted into the movie table and if it isn't, the operation is skipped. This safeguard prevents the insertion of malformed JSON data.
Let’s consider another example combining the two rules, the constraint in the creation phase of the movie table, and the json_valid()
check in the insertions. Considering the following query:
INSERT INTO movie ( data ) SELECT
'{"Year":"1979"}' AS movie_input
WHERE
json_valid ( movie_input );
The result of this query would be a “CHECK constraint failed” error message since the input value doesn’t have a Name field and the Year field is not an integer, so the insert would fail, even though the provided JSON data is a valid JSON.
Moreover, for better thorough schema validation over complicated and nested JSON data, you may consider Python's JSONschema library as well.
Section 5: How To Manage Nested JSON Data in SQLite
Navigating nested and hierarchical JSON data in SQLite can present some challenges. However, SQLite's inbuilt JSON functions streamline this process and make it manageable. Here you can see some strategies to manage nested JSON in SQLite.
Unfolding Hierarchical JSON Data Using SQL Querying
SQLite's json_each()
and json_extract()
functions can help you navigate through the layers of nested JSON data. Consider this query that uses json_each()
to parse through the data and json_extract()
to selectively pull the required information.
For example, this query will dig into the Cast array in each JSON record in the data
field in the movie
table, and will list the movies
that have more than 2 Cast
members:
SELECT
key,
json_extract ( data, '$.Name' ) AS movie_name,
json_extract ( data, '$.Year' ) AS movie_year,
json_array_length ( json_extract ( data, '$.Cast' ) ) AS cast_size
FROM
movie,
json_each ( data )
WHERE
type = 'array'
AND cast_size > 2
GROUP BY
movie_name;
The result of the above query would be something like this:
key | movie_name | movie_year | cast_size |
---|---|---|---|
Simone Mikey Bryn | Forgotten in the Planet | 1970 | 3 |
Navigating Through JSON Arrays by SQL Querying
JSON objects can hold important information in the form of an array, by using json_tree()
and json_extract()
in combination, you can iterate through these nested arrays and extract data from them.
For example, this query fetches each Actor
’s name from the Cast array of each movie record:
SELECT
json_extract ( data, je.fullkey ) AS actor,
json_extract ( data, '$.Name' ) AS movie_name,
json_array_length ( data, '$.Cast' ) AS cast_size
FROM
movie,
json_tree ( data ) AS je
WHERE
( je.type = 'text' )
AND ( je.fullkey LIKE '%Cast%' );
The result of this query would be this:
actor | movie_name | cast_size |
---|---|---|
Adrian Gratianna | Forgotten in the Planet | 3 |
Tani O'Hara | Forgotten in the Planet | 3 |
Tessie Delisle | Forgotten in the Planet | 3 |
Dacy Dex Elsa | The Obsessed's Fairy | 2 |
Matilde Kenton Collins | The Obsessed's Fairy | 2 |
Margery Maximilianus Shirk | Last in the Kiss | 2 |
Harri Garwood Michelle | Last in the Kiss | 2 |
Adrian Gratianna | Forgotten in the Planet | 3 |
Tani O'Hara | Forgotten in the Planet | 3 |
Tessie Delisle | Forgotten in the Planet | 3 |
Flattening JSON Data Using the json_each()
Function in SQLite
At times, simplifying nested JSON structures by flattening can be a practical approach to solving some complex queries against JSON objects. SQLite's json_tree()
function can be used for flattening JSON objects.
For example, this query employs json_tree()
to convert the JSON data into a table of key-value pairs, completely flattened, the query would fetch each primary value type, going through arrays and objects as well, of the first movie record:
SELECT
jt.fullkey,
jt.key,
jt.value
FROM
movie,
json_tree ( data ) AS jt
WHERE
( jt.key<> '' )
AND ( jt.type IN ( 'integer', 'text', 'real' ) )
AND json_extract ( data, '$.ID' ) = 1
The result of this query would be this:
fullkey | key | value |
---|---|---|
$.ID | ID | 1 |
$.Name | Name | Forgotten in the Planet |
$.Year | Year | 1970 |
$.Genre[0] | 0 | Comedy |
$.Genre[1] | 1 | Crime |
$.Director | Director | Henrie Randell Githens |
$.Cast[0] | 0 | Adrian Gratianna |
$.Cast[1] | 1 | Tani O'Hara |
$.Cast[2] | 2 | Tessie Delisle |
$.Runtime | Runtime | 90 |
$.Rate | Rate | 7 |
By adopting these methods, you can efficiently parse, manage, and decode JSON data in SQLite, which is invaluable when dealing with complex JSON data.
Section 6: How To Use Indexing for Query Optimization Over JSON Data in SQLite?
Indexing JSON data in SQLite is an effective way to optimize search operations and enhance query performance, especially for large datasets. By creating an index based on certain JSON properties, you can significantly expedite search operations on a JSON column.
The principle behind this approach is simple. Instead of performing a full table scan and parsing the JSON for each row, which can be resource-consuming, SQLite can leverage the index to quickly locate the rows of interest.
How To Add SQL Indexing on JSON Data in SQLite?
Let's consider a practical example with the movie
dataset. For instance, if you frequently search for movies by their Name
, creating an index on this property would be beneficial:
CREATE INDEX idx_name ON movie ( json_extract ( data, '$.item.Name' ) );
Here, the data
is the column with the JSON data, and the movie
is the table. The json_extract()
function extracts the Name
of each movie
's JSON data, and SQLite uses this value to create an index.
Once you run this query and the index is established, SQLite can quickly retrieve data when you query for a movie by its Name
. This query would be much faster with the idx_name index in place. Therefore, adding indexing to JSON data in SQLite offers powerful optimization capabilities, making it an efficient way to manage large JSON datasets.
How To Create One Index on Multiple Fields of JSON Data in SQLite?
Let's consider another example in which you may query for specific data more often based on more than 1 field. For example, if you frequently search for movies
by Name and Year, creating an index on these properties together would be beneficial. In SQLite, this could be done by creating an index on a calculated expression:
CREATE INDEX idx_name_year ON movie ( json_extract ( data, '$.Item.Name' ), json_extract ( data, '$.Item.Year' ) );
Once again, when this index is established, SQLite can quickly retrieve data when you query for a movie by Name and Year.
Section 7: Json5 Support in SQLite
The JSON5 was introduced to support some ECMA-compatible syntax and make JSON a bit more fit to be used as a configuration language. SQLite introduced the JSON5 extension support in version 3.42.0. While SQLite can read and interpret JSON text that includes JSON5 extensions, any JSON text SQLite’s functions generate will strictly fit the definition of canonical JSON. Here are some of the primary features the JSON5 extension adds to JSON support in SQLite.
JSON Objects With Comments in SQLite JSON
JSON5 allows for single (//...) and multi-line (/.../) comments. This can be particularly useful for adding context or explanations directly within your JSON data. Here’s an example of comments in JSON objects:
/* A
multi-line
comment
in JSON5 */
{
"key": "value" // A single-line comment in JSON5
}
Object Keys Without Quotes in SQLite JSON
In JSON5, object keys can be unquoted identifiers, simplifying your JSON syntax. However, it's important to note that this may limit compatibility with systems strictly following JSON standards.
{
key: "value"
}
Multiline Strings in JSON Objects
JSON5 supports multiline strings, which can be achieved by escaping new line characters. This is useful when dealing with large strings or when formatting the string in a more readable format.
{
key: "This is a \\\\\\\\
multiline string"
}
Json5 vs Canonical JSON Validation in SQLite
Here we’ll be going through the complete validation techniques for JSON5 and canonical JSON objects, explaining their support by precise SQL query examples in the SQLite database.
To determine whether a string is valid JSON5, you can use the json_error_position()
function. This function will return a non-zero value if the string is not well-formed JSON or JSON5. Here’s an example:
SELECT
json_error_position ( '{ key: "value"}' ) AS error_position;
The result of this query would be 0 indicating that no error is detected here, even though the key is unquoted since this is a valid extension of JSON5.
error_position |
---|
0 |
On the other hand, to convert a JSON5 string into canonical JSON, you can use the json()
function. While this function recognizes and processes JSON5 input, it will output canonical JSON only. This allows for backward compatibility with systems expecting canonical JSON. Here’s an example:
SELECT
JSON ( '{key: "value"}' ) AS canonical_json;
The result of this query would be a canonical JSON, converted from the JSON5 format, which made the key quoted here:
canonical_json |
---|
{"key": "value"} |
However, be aware that the json_valid()
function will continue to report false for inputs that are not canonical JSON, even if the input is valid JSON5. This is an important distinction when working with both canonical JSON and JSON5 in SQLite. For example, consider the following query:
SELECT
json_valid ( '{key: "value"}' ) AS valid_json;
The result of this query would be 0 indicating that this is not a valid JSON, since it has an unquoted key which is a violation of canonical JSON format:
valid_json |
---|
{"key": "value"} |
Section 8: Common Mistakes and Troubleshooting When Working With JSON in SQLite
Handling JSON data in SQLite involves some common pitfalls that can be avoided with a deeper understanding of the specific mechanisms, such as the correct use of functions. Here are some key considerations.
How To Debug Syntax Errors in JSON Data in the JSON Parsing Phase of SQLite?
JSON data must be formatted correctly and follow a specific standard syntax to be parsed and processed in the SQLite database. If your JSON string is improperly formatted, SQLite won't be able to interpret it, resulting in errors. For example, you may have mismatched brackets, incorrect use of quotes, or misplaced commas.
SQLite provides the json_valid()
function for validating JSON string, as the name suggests. json_valid()
function returns 1 if the input is a well-formed JSON string and 0 otherwise. Here's an example:
SELECT json_valid('{"Name":"Naked of Truth","Year":1979}');
In the case of a syntax error in the JSON string, the json_error_position()
function can be used to identify the position in the string where the error happened:
SELECT json_error_position('{"Name":"Naked of Truth","Year":1979}');
Incorrect Use of JSON Functions While Querying Against JSON Data
Misuse of JSON functions is another common issue, so ensuring a solid understanding of the JSON functions and their usage in SQLite is crucial for successful data handling. For instance, using the wrong path or failing to account for the zero-based index system of JSON arrays in SQLite can lead to errors or incorrect data retrievals.
No BLOB Support in SQLite’s JSON Functions
It's important to ensure you're not attempting to use BLOBs with JSON functions in SQLite because all of the JSON functions in SQLite currently throw an error if any of their arguments are BLOBs and not valid JSON as input. SQLite currently does not support any binary encoding of JSON, while this is a potential future enhancement.
How To Do JSON Validation While SQL Querying JSON Data in SQLite?
The json()
function in SQLite is primarily used to enforce the JSON formatting of a string by adding quotes, escaping necessary characters, etc. Using the json()
function incorrectly could result in a lack of error-catching and potential data inconsistencies.
However, it's not designed to validate a JSON. For validating a JSON string or finding a syntax error, use the json_valid()
and json_error_position()
functions as discussed previously.
Wrapping Up
In this comprehensive guide, we've journeyed through the powerful integration of JSON and SQLite, offering insight into the vast opportunities this combination provides. We started with an overview of SQLite's JSON functions along with their detailed use cases with SQL query examples.
We explored advanced querying techniques like handling hierarchical JSON data within SQLite. The journey deepened into the mechanics of decoding and managing JSON data, highlighting the usefulness of SQLite functions like json_each()
and json_tree()
. We also addressed the value of flattening JSON data for efficient data handling.
Then we moved into a significant area that is often overlooked, performance-boosting via indexing. This powerful optimization can greatly speed up query performance and enhance your SQLite experience with JSON. The new-age JSON5 extension was then discussed, bringing more flexibility to your JSON data formatting.
Lastly, we addressed some common mistakes and troubleshooting tips to smoothen your journey through JSON in SQLite, reinforcing the importance of correct JSON syntax and the proper use of SQLite JSON functions.
Remember, learning and experimentation are the keys to unlocking the full potential of JSON in SQLite. As you apply these techniques to your projects, do share your experiences to help others on a similar journey. So, let's continue learning and pushing boundaries with JSON in SQLite. Have a good JSON use!